fastjson
https://repo1.maven.org/maven2/com/alibaba/fastjson/1.2.24/
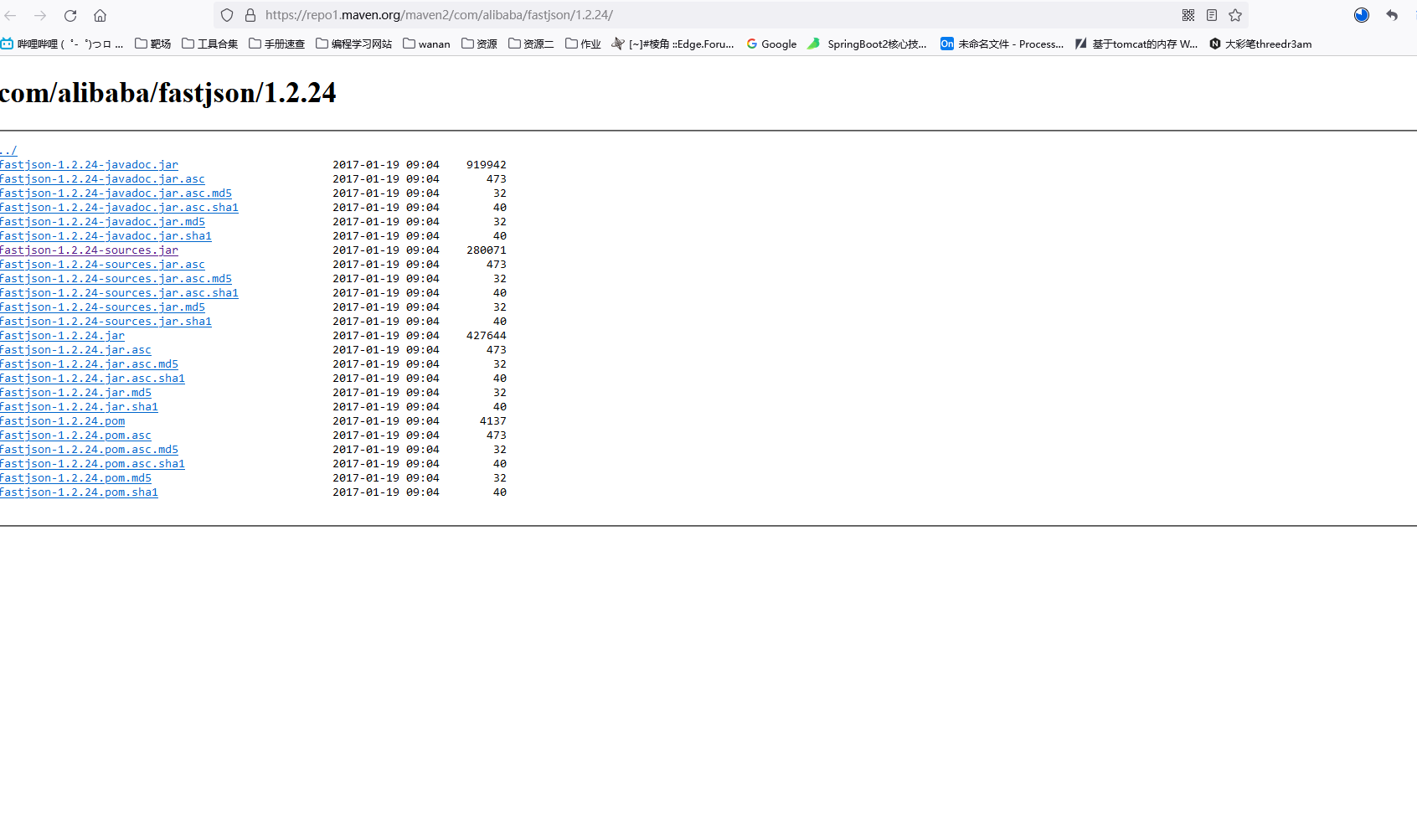
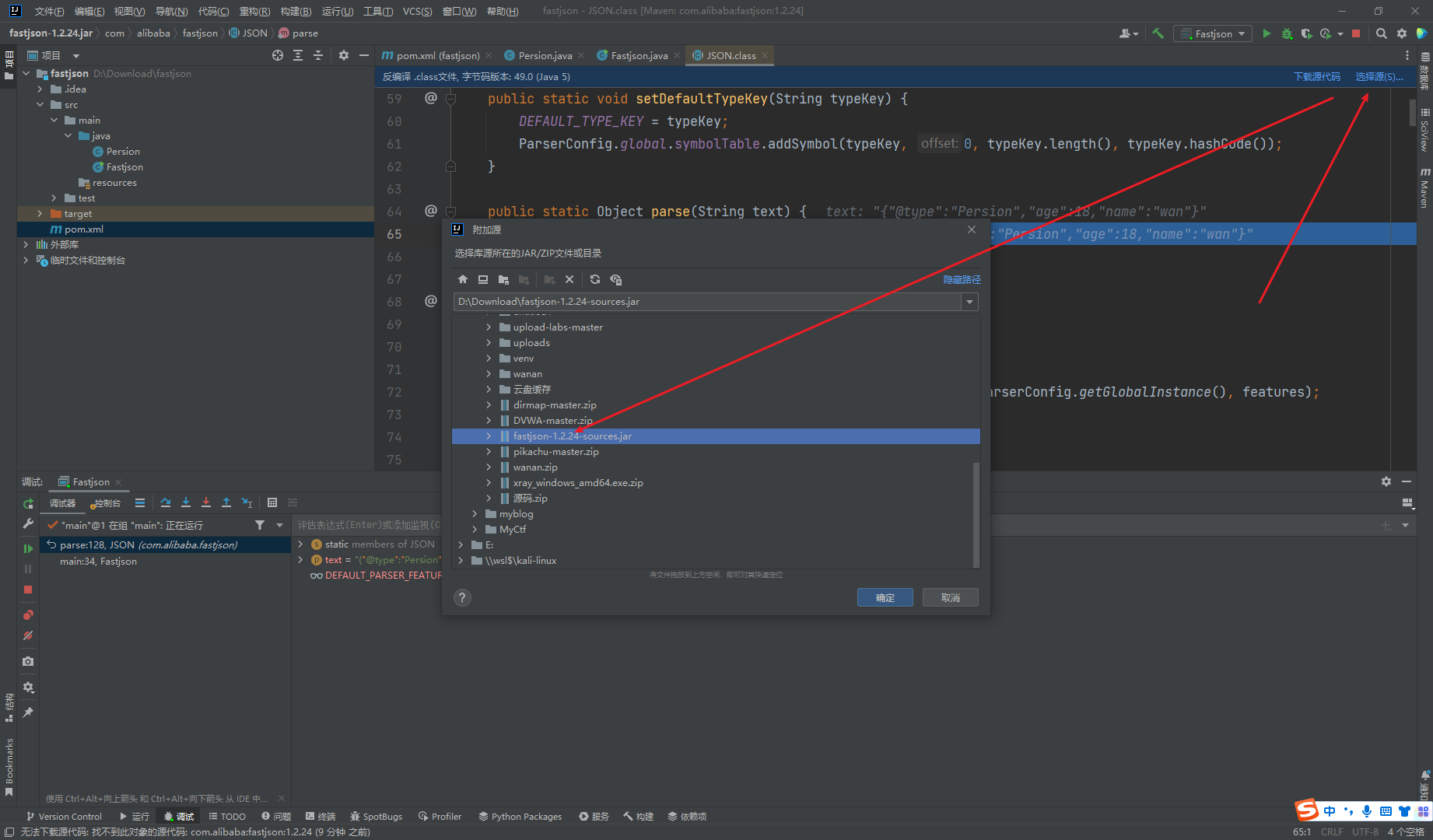
<dependencies> <dependency> <groupId>com.alibaba</groupId> <artifactId>fastjson</artifactId> <version>1.2.24</version> </dependency> </dependencies>
|
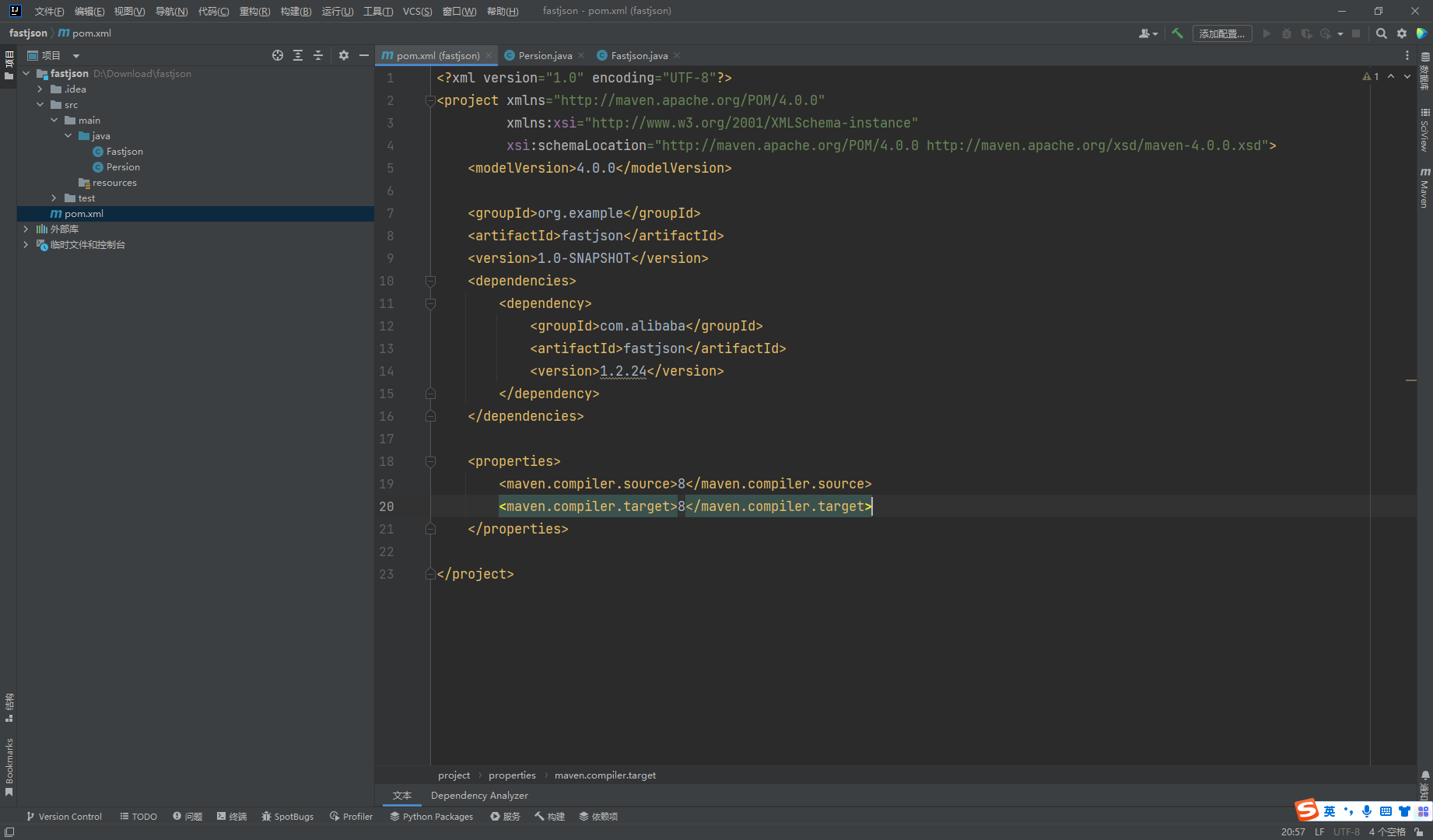
Persion.java
public class Persion { public String name; private int age;
@Override public String toString() { return "Persion{" + "name='" + name + '\'' + ", age=" + age + '}'; }
public String getName() {
return name; }
public void setName(String name) {
this.name = name; }
public int getAge() {
return age; }
public void setAge(int age) {
this.age = age; }
public Persion() { }
public Persion(String name, int age) { this.name = name; this.age = age; } }
|
Fastjson.java
import com.alibaba.fastjson.JSONObject; import com.alibaba.fastjson.serializer.SerializerFeature;
import java.sql.SQLOutput;
public class Fastjson { public static void main(String[] args) throws Exception{
Persion persion = new Persion("wan", 18);
String s = JSONObject.toJSONString(persion);
System.out.println("s:" + s);
String s1 = JSONObject.toJSONString(persion, SerializerFeature.WriteClassName); System.out.println("s1:"+ s1);
} }
|
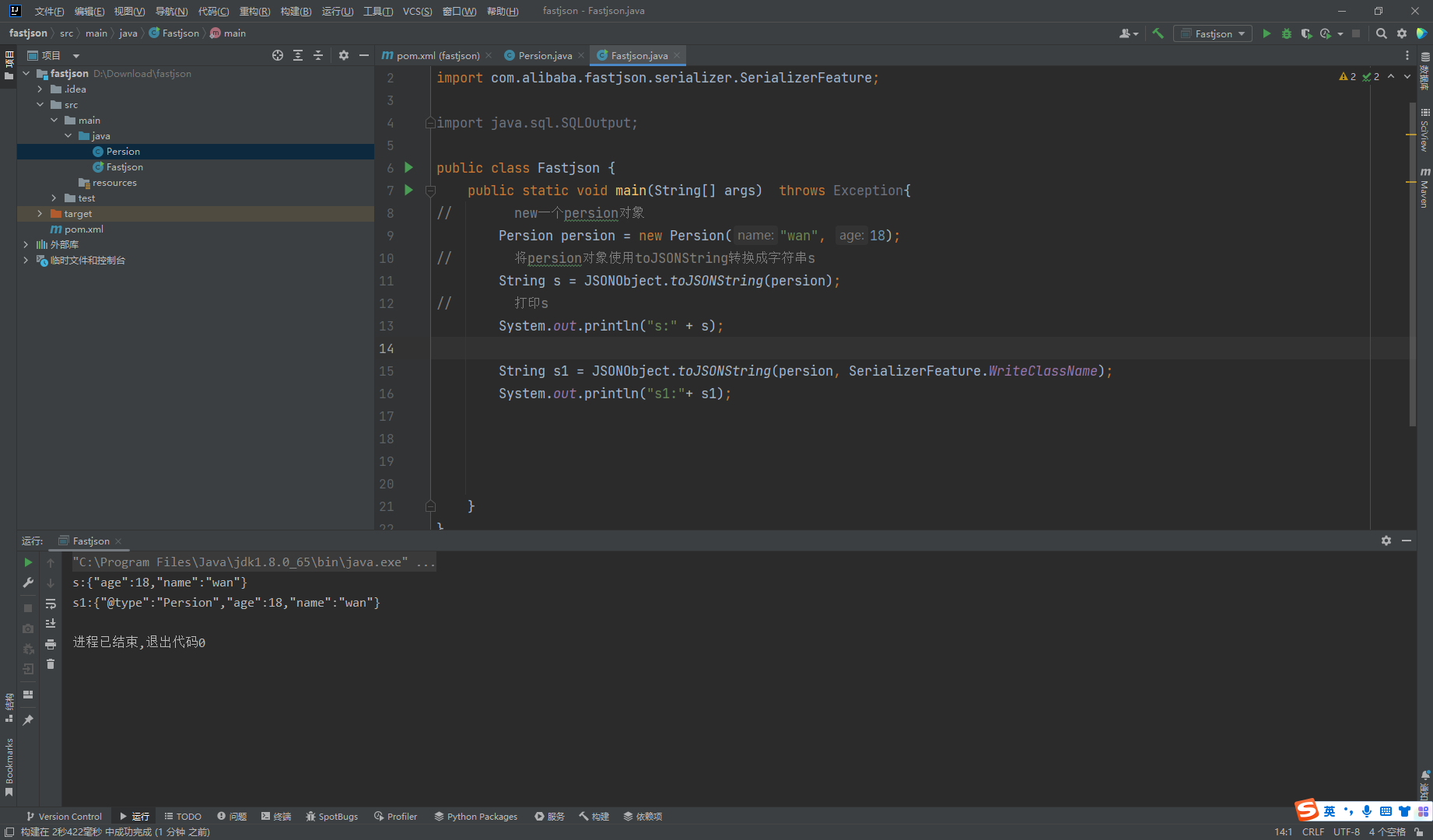
import com.alibaba.fastjson.JSONObject; import com.alibaba.fastjson.serializer.SerializerFeature;
import java.sql.SQLOutput;
public class Fastjson { public static void main(String[] args) throws Exception{
Persion persion = new Persion("wan", 18);
String noType = JSONObject.toJSONString(persion);
System.out.println("noType:" + noType);
String type = JSONObject.toJSONString(persion, SerializerFeature.WriteClassName); System.out.println("type:"+ type);
String noType1 = "{\"age\":18,\"name\":\"wan\"}";
Object noType1parse = JSONObject.parse(noType1); System.out.println(noType1parse);
JSONObject noType1Object = JSONObject.parseObject(noType1); System.out.println(noType1Object);
String type1 = "{\"@type\":\"Persion\",\"age\":18,\"name\":\"wan\"}";
Object type2parse = JSONObject.parse(type1); System.out.println(type2parse);
JSONObject type2Object = JSONObject.parseObject(type1); System.out.println(type2Object);
} }
|
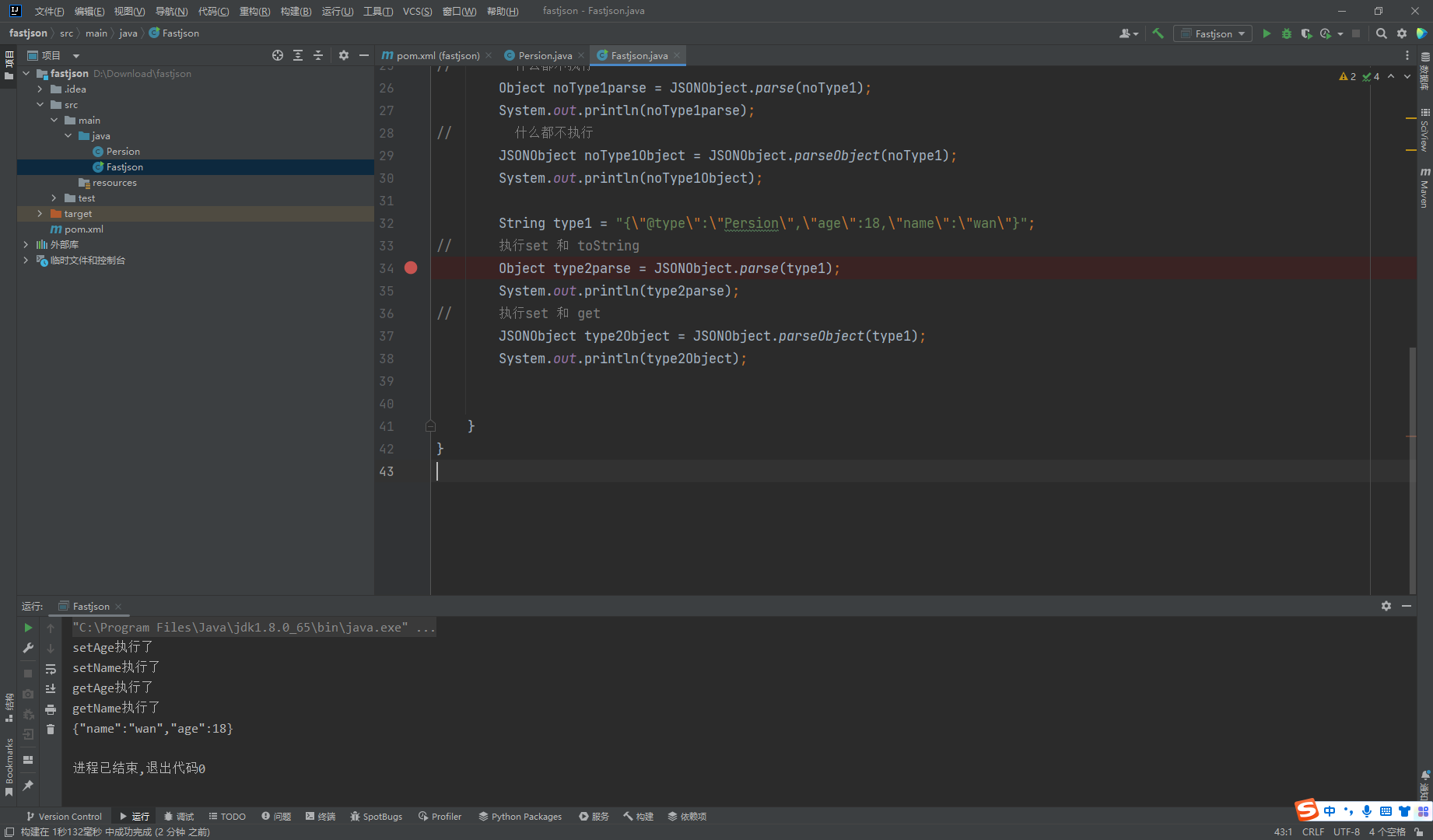
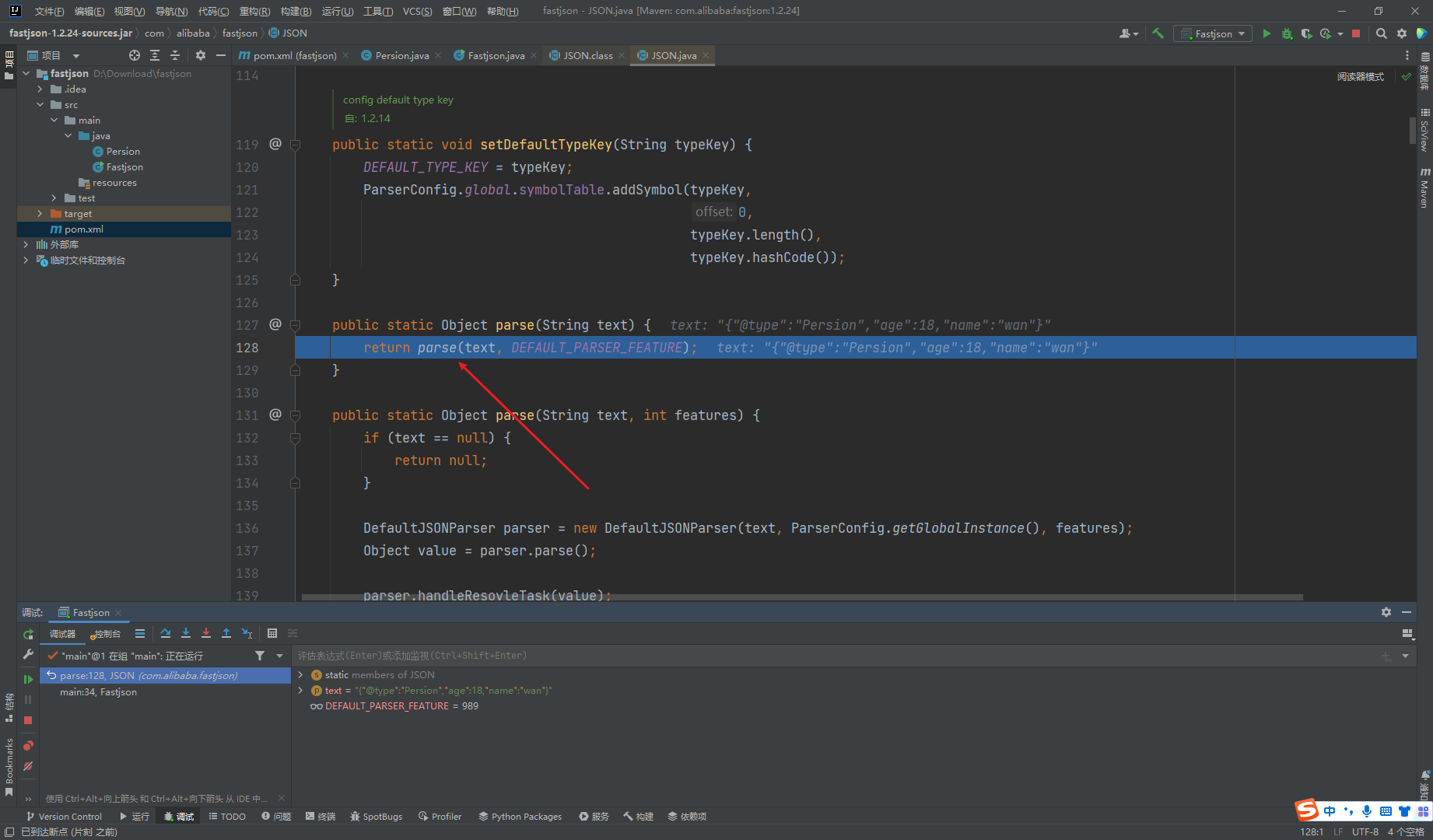
这里调了parse
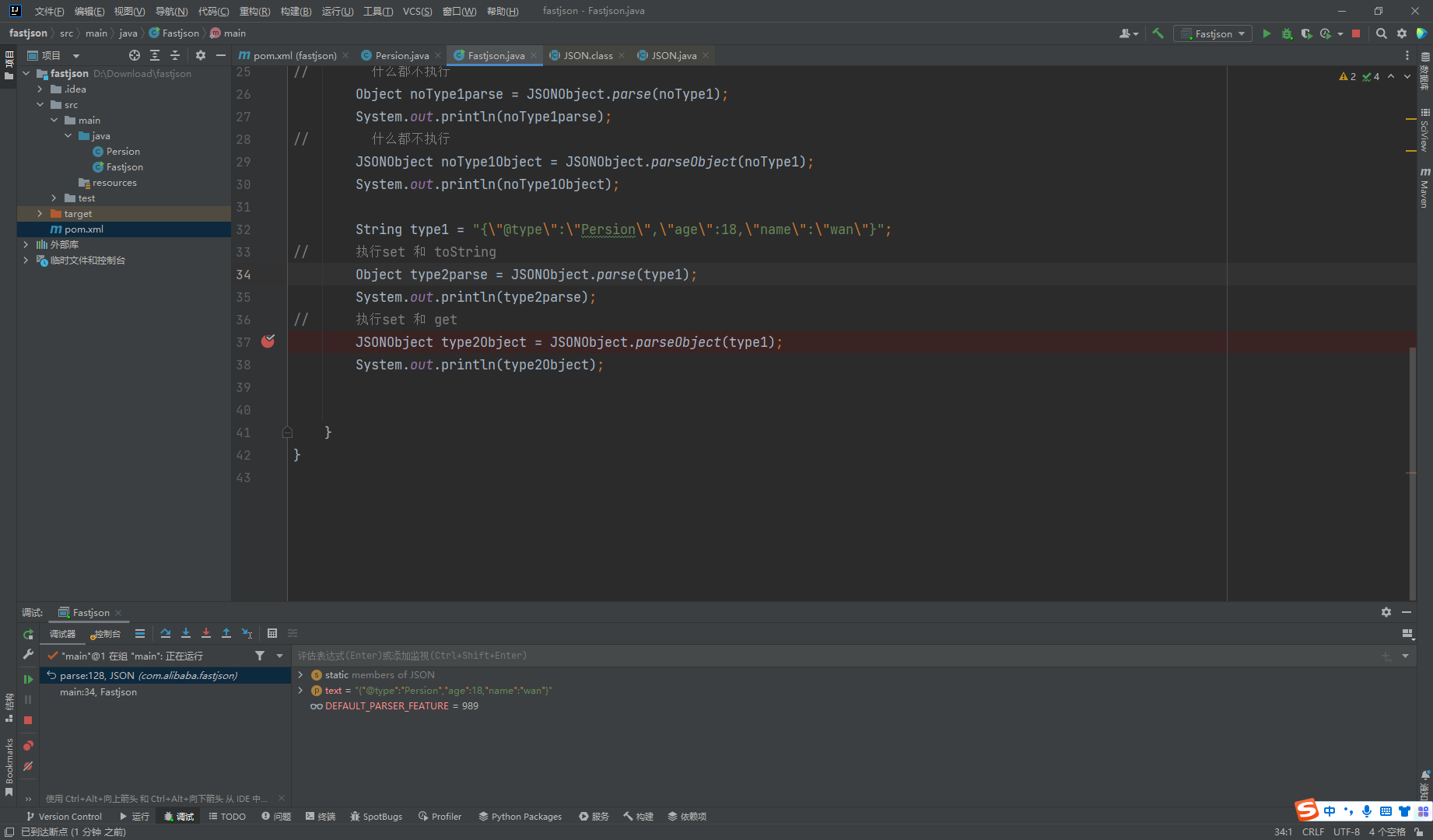
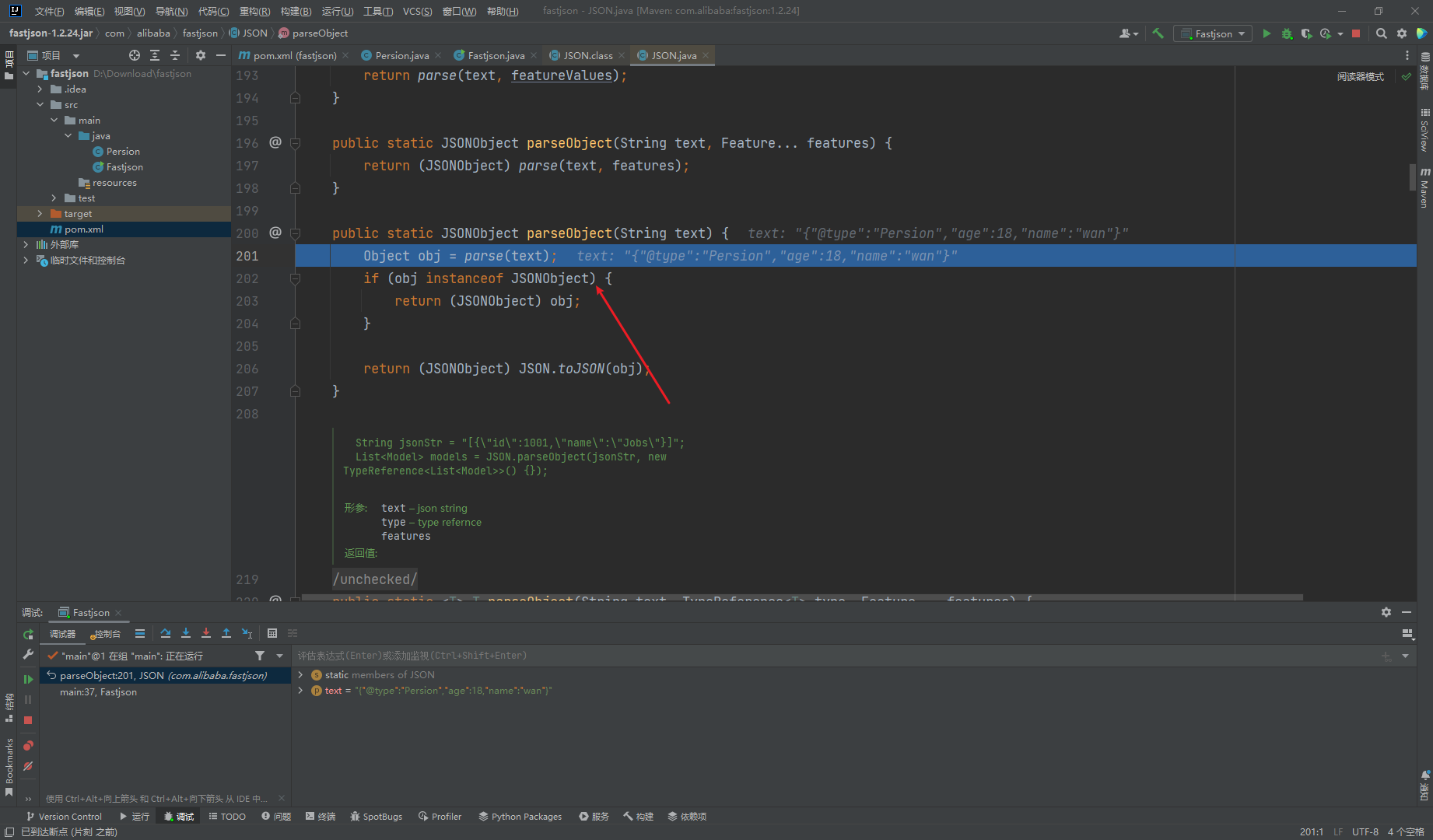
这里多了一个转换成json对象
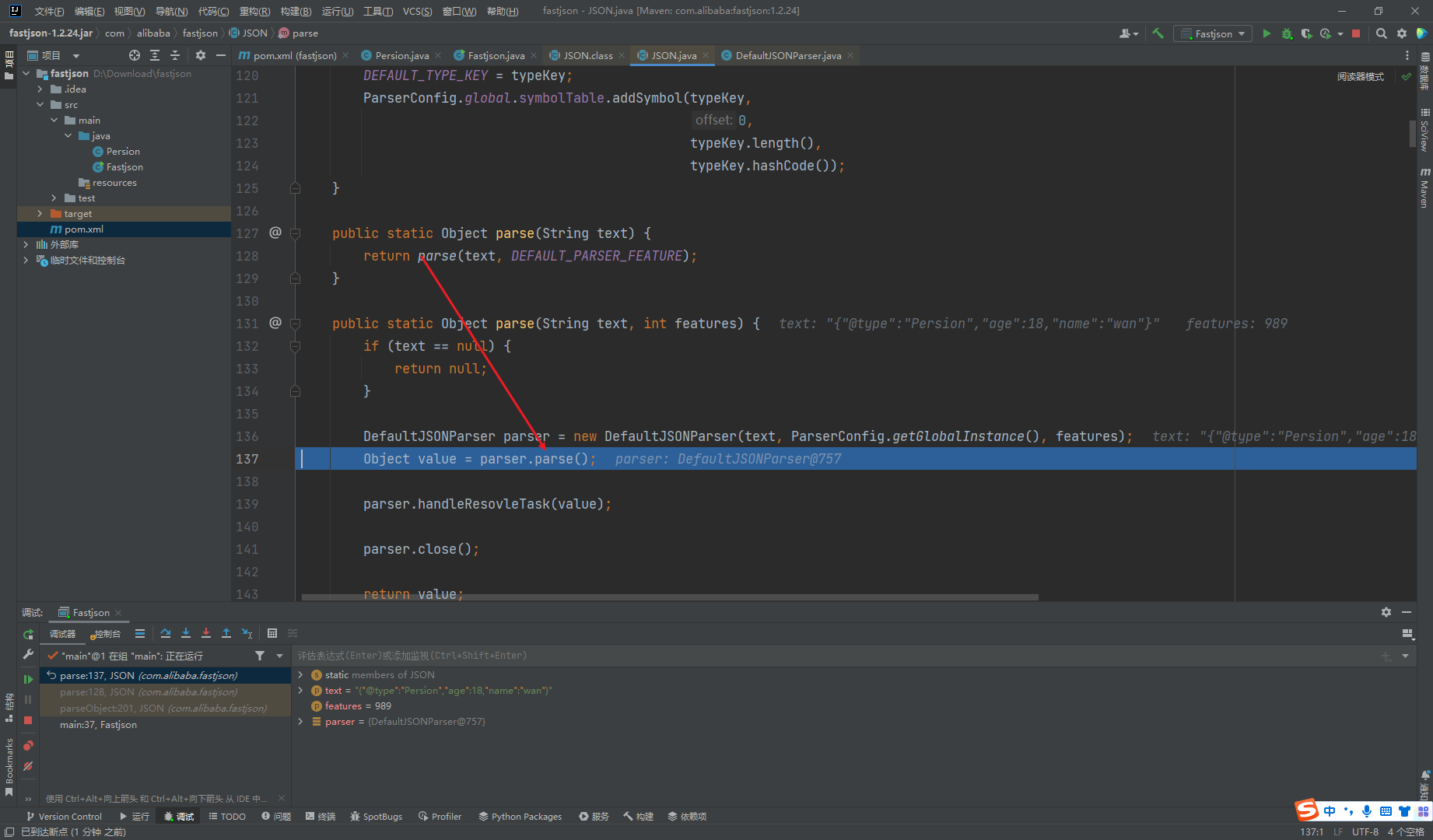
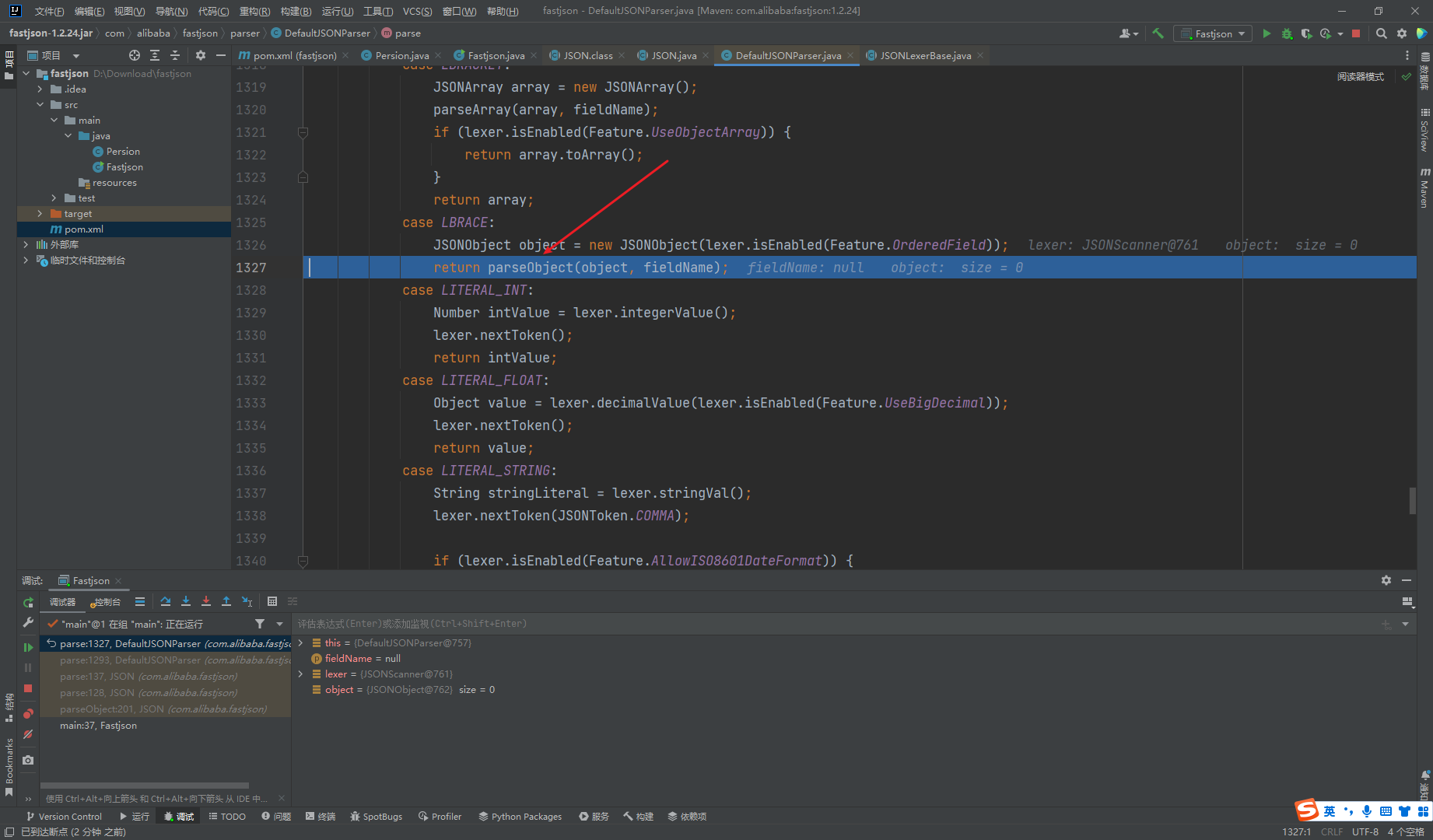
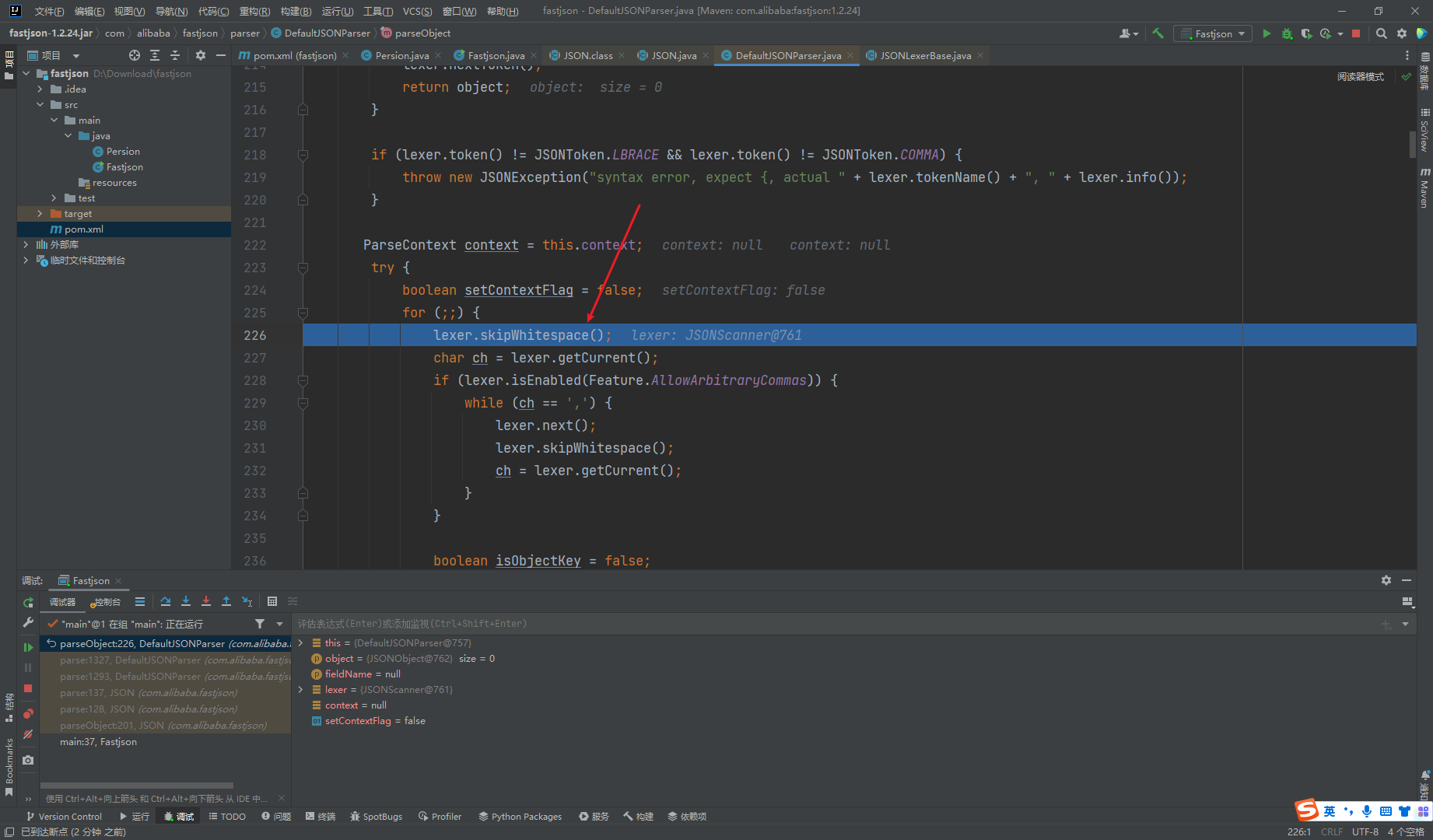