TowSum
给定一个整数数组 nums 和一个整数目标值 target,请你在该数组中找出 和为目标值 target 的那 两个 整数,并返回它们的数组下标。
- 创建一个map
- for循环遍历nums数组
- 用target - nums[i],用来计算那个数跟当前的数字相加得到target
- 检查map中是否有这个数,如果有则返回结果,如果没有则把num[i]当做key,i当做value放入map中
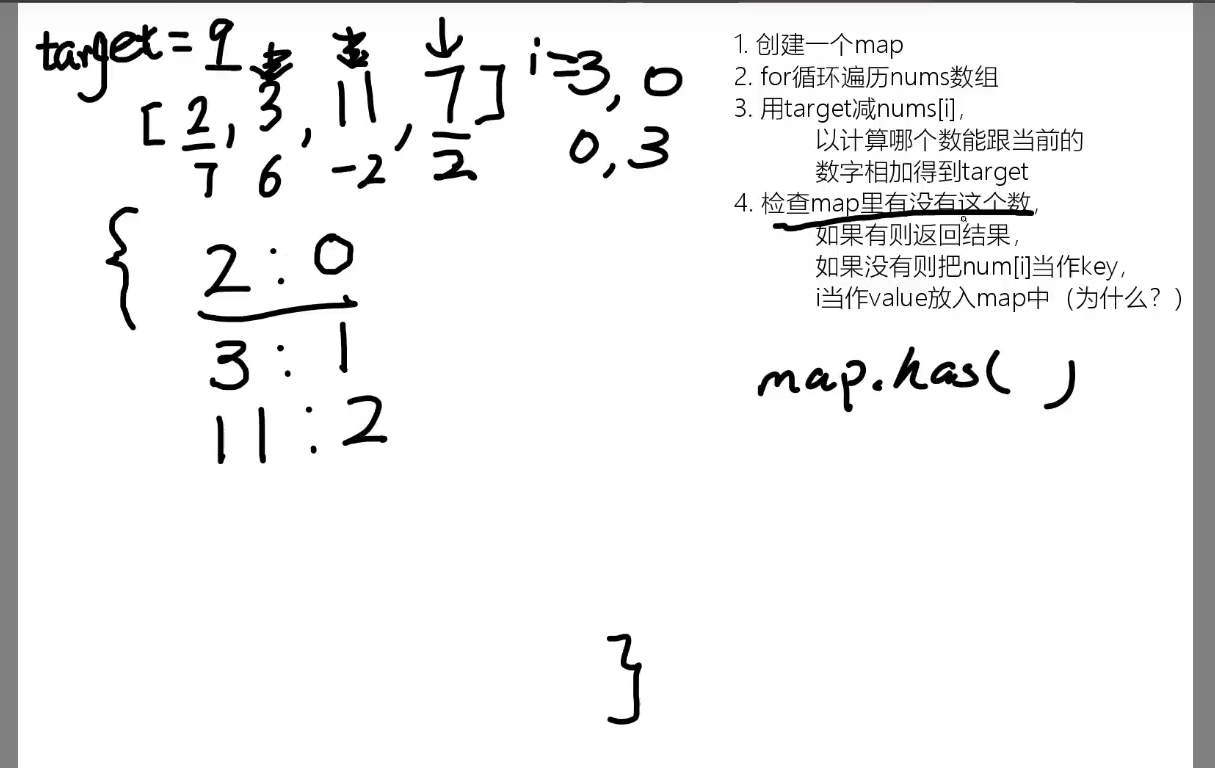
package wanan;
import java.util.HashMap; import java.util.Map;
public class TwoSum { public int[] twoSum(int[] nums,int target){
HashMap<Integer, Integer> map = new HashMap<>();
for(int i = 0;i<nums.length;i++){
int complement = target - nums[i];
if (map.containsKey(complement) && map.get(complement) != i){
return new int[]{i, map.get(complement)};
}else { map.put(nums[i],i);
} }
return new int[0];
} }
|
AddTwoNumbers
给你两个 非空 的链表,表示两个非负的整数。它们每位数字都是按照 逆序 的方式存储的,并且每个节点只能存储 一位 数字。
请你将两个数相加,并以相同形式返回一个表示和的链表。
你可以假设除了数字 0 之外,这两个数都不会以 0 开头。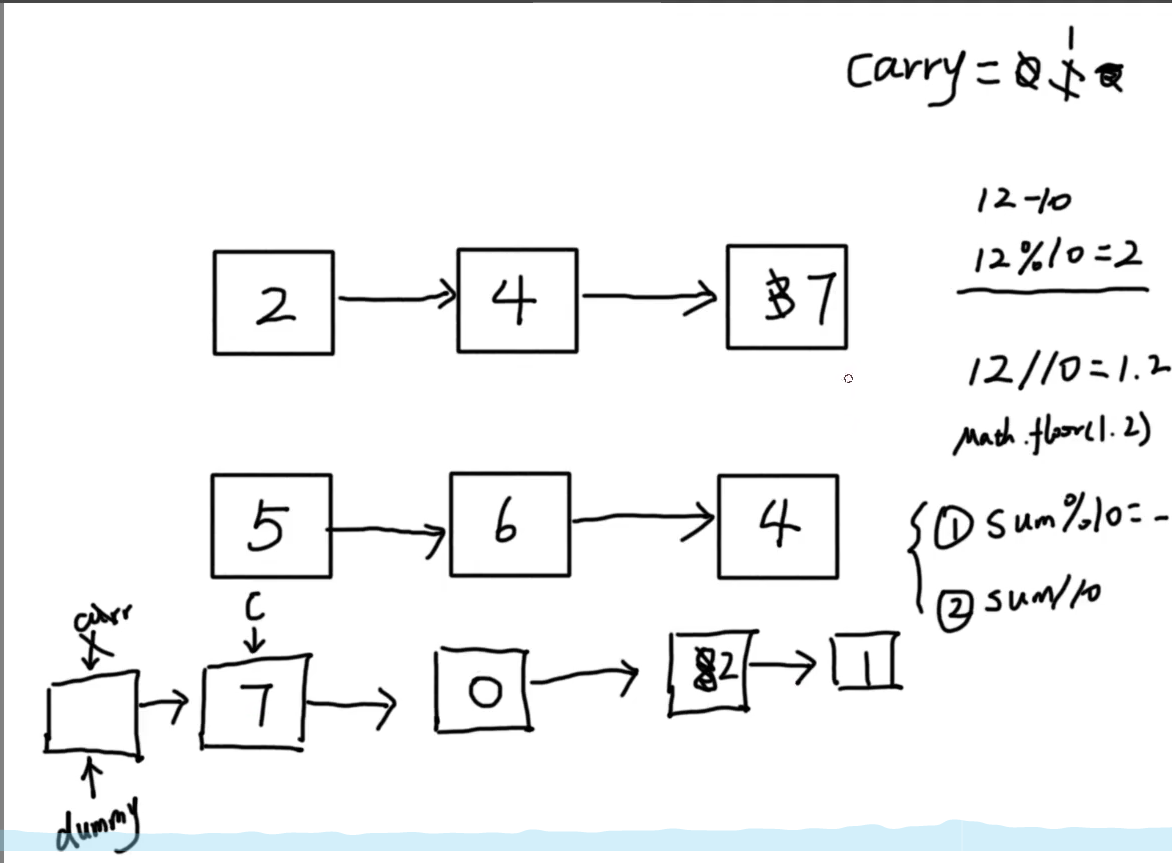
package wanan; class ListNode{ public int val;
public ListNode next = null;
public ListNode(int val){ this.val = val; } }
public class AddTwoNumbers { public ListNode addTwoNumbers(ListNode l1,ListNode l2){
ListNode dummy = new ListNode(0);
ListNode curr = dummy;
int carry = 0;
while(l1 != null || l2 != null){
int sum = 0;
if(l1 != null){ sum += l1.val;
l1 = l1.next;
} if (l2 !=null){ sum += l2.val;
l2 = l2.next;
} sum += carry; curr.next = new ListNode(sum % 10);
carry = sum / 10;
curr = curr.next;
}
if (carry > 0){ curr.next = new ListNode(carry); } return dummy.next; } }
|
LengthOfLongestSubstring
给定一个字符串 s ,请你找出其中不含有重复字符的 最长子串 的长度。
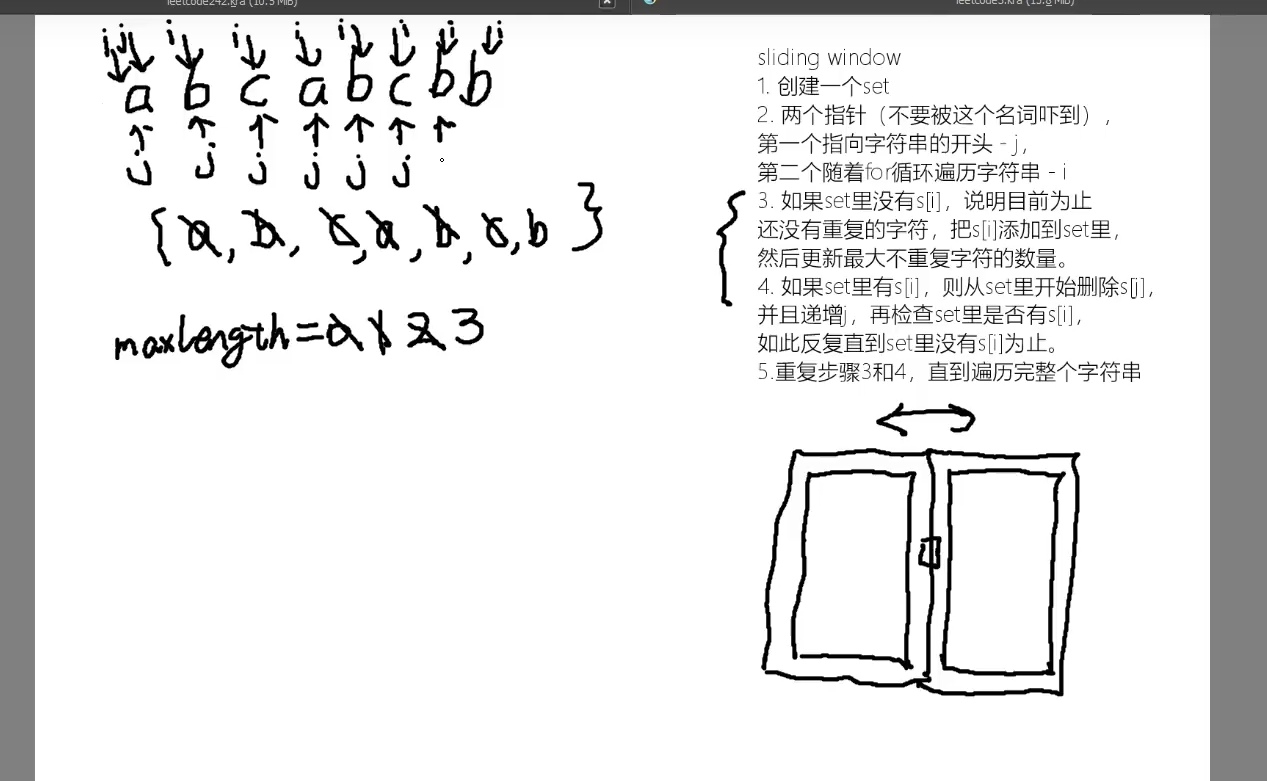
package wanan;
import java.util.HashSet;
public class LengthOfLongestSubstring { public int lengthOfLongestSubstring(String s){
HashSet<Character> set = new HashSet<>();
int j = 0,maxLength = 0;
if(s == null|| s.length() == 0){
return 0; } for(int i= 0;i<s.length();i++){ if (!set.contains(s.charAt(i))){
set.add(s.charAt(i)); maxLength = Math.max(maxLength, set.size());
}else { while (set.contains(s.charAt(i))){
set.remove(s.charAt(j)); j++;
}
set.add(s.charAt(i)); } } return maxLength; } }
|
LongestPalindromicSubstring
给你一个字符串 s,找到 s 中最长的回文子串。
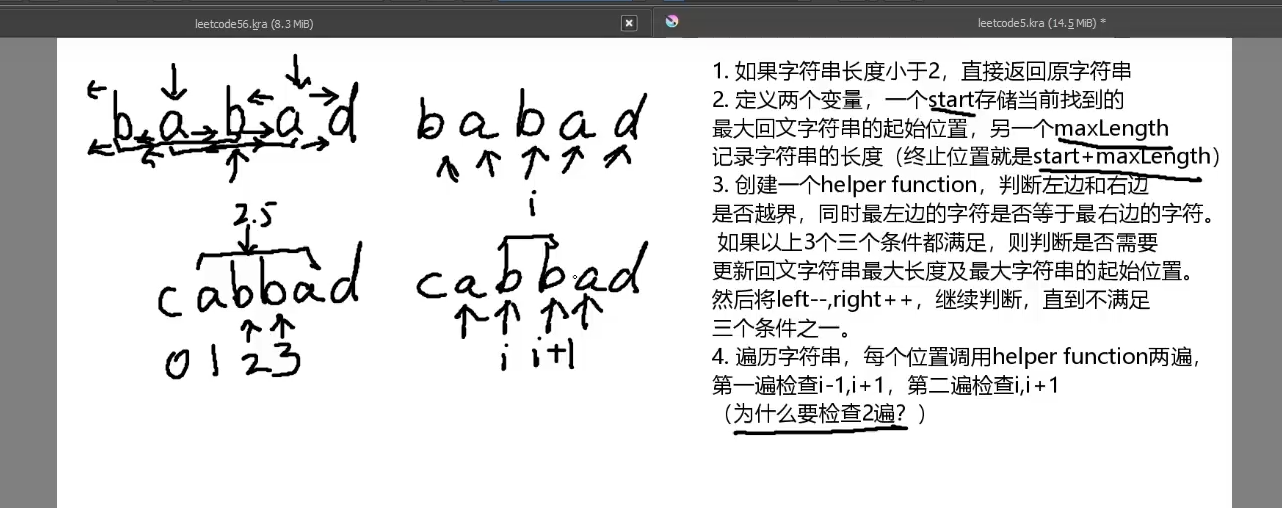
package wanan;
class LongestPalindromicSubstring{ private int start =0 ,maxLength = 1;
public String longestPalindrome(String s){ if (s.length() < 2){
return s; }
for (int i= 0;i <s.length();i++){
expandAroundCenter(s,i -1,i+1); expandAroundCenter(s,i ,i+1); } return s.substring(start,start+maxLength);
} private void expandAroundCenter(String s,int left,int right){
while (left >= 0 && right < s.length() && s.charAt(left) == s.charAt(right)){
if (right - left +1 > maxLength){
maxLength = right - left +1; start = left; } left -- ; right ++;
} }
}
|
ThreeSum
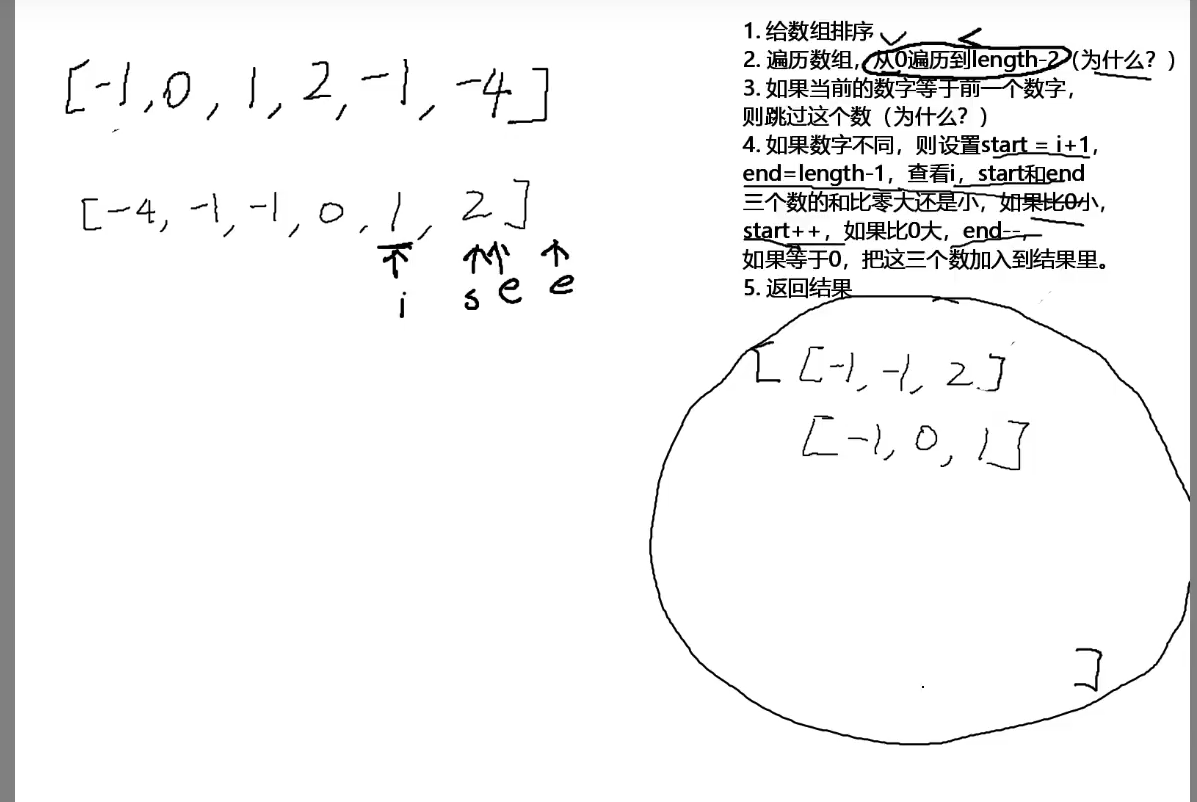
package wanan;
import java.util.ArrayList; import java.util.Arrays; import java.util.List;
class ThreeSum{ public List<List<Integer>> threeSum(int[] nums){
ArrayList<List<Integer>> result = new ArrayList<>(); Arrays.sort(nums);
for (int i=0 ;i < nums.length -2 ;i++){
if ( i== 0 || nums[i] != nums[i - 1]){
int start = i +1 , end = nums.length - 1;
while (start < end) {
if (nums[i] + nums[start] + nums[end] == 0) {
result.add(Arrays.asList(nums[i], nums[start], nums[end]));
start++; end--;
while (start < end && nums[start] == nums[start - 1]) { start++; }
while (start < end && nums[end] == nums[end + 1]) { end--; }
} else if (nums[i] + nums[start] + nums[end] < 0) { start++; } else { end--; } } } } return result; } }
|